运行原理(初步)
SpringBoot的运行需要从pom.xml文件开始探究。
1. 父依赖
pom.xml中有一个父依赖spring-boot-starter-parent
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.4.1</version> <relativePath/> <!-- lookup parent from repository --> </parent>
而这个依赖下,还有一个父依赖spring-boot-dependencies
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>2.4.1</version> </dependency>
- spring-boot-dependencies:核心依赖在父工程中。
- spring-boot-dependencies中有版本仓库。我们在引入一部分依赖的时候,不需要指定版本,因为SpringBoot会根据当前的版本去指定一些依赖的版本,我们只需要导入依赖即可,maven会从父工程中查找对应的版本号。
spring-boot-dependencies是SpringBoot的版本控制中心,管理应用中所有依赖版本的地方。如果此依赖已经定义要导入依赖的版本,就不需要手动添加版本。
2. 启动器spring-boot-starter
样式如下
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-xxx</artifactId>
</dependency>
springboot-boot-starter-xxx,说白了就是Springboot的启动场景
比如spring-boot-starter-web,他就会帮我们自动导入web的所有依赖
springboot会将所有的功能场景,都变成一个个的启动器
我们要使用什么功能,就只需要找到对应的启动器就好了
3. 主程序
【挖坑】这里比较混乱,其实原理还没搞懂。待以后懂了再做
探究路径
@SpringBootApplication
├── @ComponentScan
├── @EnableAutoConfiguration
│ ├── @AutoConfigurationPackage
│ │ └── @Import({Registrar.class})
│ └── @Import({AutoConfigurationImportSelector.class})
│ └── AutoConfigurationImportSelector.class
│ ├── List<String>\ configurations\ =\ this.getCandidateConfigurations(annotationMetadata,\ attributes);
│ └── protected\ List<String>\ getCandidateConfigurations(AnnotationMetadata\ metadata,\ AnnotationAttributes\ attributes)
│ ├── SpringFactoriesLoader.loadFactoryNames()
│ │ └── \ private\ static\ Map<String,\ List<String>>\ loadSpringFactories(ClassLoader\ classLoader)
│ │ └── loadSpringFactories
│ │ ├── private\ static\ Map<String,\ List<String>>\ loadSpringFactories(ClassLoader\ classLoader)
│ │ └── public\ static\ final\ String\ FACTORIES_RESOURCE_LOCATION\ =\ "META-INF:spring.factories";
│ └── this.getSpringFactoriesLoaderFactoryClass()
└── @SpringBootConfiguration
└── @Configuration
└── @Component
3.1. 默认的主启动类
//用来标注一个程序的主程序类,说明这是一个Spring Boot应用
@SpringBootApplication
public class MyspringbootApplication {
public static void main(String[] args) {
//启动一个服务
//该方法返回一个ConfigurableApplicationContext对象
//参数一:应用入口的类; 参数二:命令行参数
SpringApplication.run(MyspringbootApplication.class, args);
}
}
3.2. @SpringBootApplication
作用:标注在某个类上,说明这个类是SpringBoot的主启动类。SpringBoot会运行这个类的main方法来启动SpringBoot应用。
进入这个注解,可以看到这个注解下还有其他注解。
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@SpringBootConfiguration
@EnableAutoConfiguration
@ComponentScan(
excludeFilters = {@Filter(
type = FilterType.CUSTOM,
classes = {TypeExcludeFilter.class}
), @Filter(
type = FilterType.CUSTOM,
classes = {AutoConfigurationExcludeFilter.class}
)}
)
public @interface SpringBootApplication {
// ...
}
3.3. @ComponentScan
组件扫描注解,对应xml配置中的元素。
作用:会自动扫描并加载符合条件的组件或者bean,将这个bean定义加载到IoC容器中。
3.4. @SpringBootConfiguration
作用:表明这是一个SpringBoot的配置类。
进入这个注解,可以看到这个注解下还有其他注解。
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Configuration
public @interface SpringBootConfiguration {
// ...
}
3.5. @Configuration
作用:表明是一个配置类
进入这个注解,可以看到这个注解下还有其他注解。
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Component
public @interface Configuration {
// ...
}
3.6. @Component
表明配置类其实是一个Spring的组件,负责启动应用。
回到@SpringBootApplication,查看其他注解。
3.7. @EnableAutoConfiguration
作用:开启自动配置功能,SpringBoot通过这个注解自动配置。
进入这个注解,可以看到这个注解下还有其他注解。
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@AutoConfigurationPackage
@Import({AutoConfigurationImportSelector.class})
public @interface EnableAutoConfiguration {
// ...
}
3.8. @AutoConfigurationPackage
作用:自动配置包。
进入这个注解,可以看到这个注解下还有其他注解。
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@Import({Registrar.class})
public @interface AutoConfigurationPackage {
// ...
}
3.9. @Import({Registrar.class})
@Import
作用:给容器导入一个组件。
Registrar.class
作用:自动配置包注册,将主启动类所在的包下面所有子包里面的所有组件扫描到Spring容器。
3.10. @Import({AutoConfigurationImportSelector.class})
作用:给容器导入组件。
AutoConfigurationImportSelector
作用:自动配置导入选择器。
打开AutoConfigurationImportSelector.class,可以看到几个配置。
// 获取所有配置
List<String> configurations = this.getCandidateConfigurations(annotationMetadata, attributes);
获得候选的配置
protected List<String> getCandidateConfigurations(AnnotationMetadata metadata, AnnotationAttributes attributes) {
List<String> configurations = SpringFactoriesLoader.loadFactoryNames(this.getSpringFactoriesLoaderFactoryClass(), this.getBeanClassLoader());
Assert.notEmpty(configurations, "No auto configuration classes found in META-INF/spring.factories. If you are using a custom packaging, make sure that file is correct.");
return configurations;
}
进入到getSpringFactoriesLoaderFactoryClass()方法
// 这里的getSpringFactoriesLoaderFactoryClass()方法
// 返回的就是我们最开始看的启动自动导入配置文件的注解类EnableAutoConfiguration
protected Class<?> getSpringFactoriesLoaderFactoryClass() {
return EnableAutoConfiguration.class;
}
getCandidateConfigurations调用了SpringFactoriesLoader类的静态方法。
进入查看SpringFactoriesLoader.loadFactoryNames()源码
这里获取所有加载的配置
public static List<String> loadFactoryNames(Class<?> factoryType, @Nullable ClassLoader classLoader) {
ClassLoader classLoaderToUse = classLoader;
if (classLoader == null) {
classLoaderToUse = SpringFactoriesLoader.class.getClassLoader();
}
String factoryTypeName = factoryType.getName();
// 这里它调用了 loadSpringFactories 方法
return (List)loadSpringFactories(classLoaderToUse).getOrDefault(factoryTypeName, Collections.emptyList());
}
点进去查看loadSpringFactories方法的类中
// 将所有的资源加载到配置类中(将下面的抽离出来分析,第15行)
public static final String FACTORIES_RESOURCE_LOCATION = "META-INF/spring.factories";
// ...
private static Map<String, List<String>> loadSpringFactories(ClassLoader classLoader) {
// 获得classLoader,我们返回可以看到这里得到的就是EnableAutoConfiguration标注的类本身
Map<String, List<String>> result = (Map)cache.get(classLoader);
if (result != null) {
return result;
} else {
HashMap result = new HashMap();
try {
// 去获取一个资源 "META-INF/spring.factories"
Enumeration urls = classLoader.getResources("META-INF/spring.factories");
// 判断有没有更多的元素,将读取到的资源循环遍历,封装成为一个Properties
while(urls.hasMoreElements()) {
URL url = (URL)urls.nextElement();
UrlResource resource = new UrlResource(url);
Properties properties = PropertiesLoaderUtils.loadProperties(resource);
Iterator var6 = properties.entrySet().iterator();
while(var6.hasNext()) {
Entry<?, ?> entry = (Entry)var6.next();
String factoryTypeName = ((String)entry.getKey()).trim();
String[] factoryImplementationNames = StringUtils.commaDelimitedListToStringArray((String)entry.getValue());
String[] var10 = factoryImplementationNames;
int var11 = factoryImplementationNames.length;
for(int var12 = 0; var12 < var11; ++var12) {
String factoryImplementationName = var10[var12];
((List)result.computeIfAbsent(factoryTypeName, (key) -> {
return new ArrayList();
})).add(factoryImplementationName.trim());
}
}
}
result.replaceAll((factoryType, implementations) -> {
return (List)implementations.stream().distinct().collect(Collectors.collectingAndThen(Collectors.toList(), Collections::unmodifiableList));
});
cache.put(classLoader, result);
return result;
} catch (IOException var14) {
throw new IllegalArgumentException("Unable to load factories from location [META-INF/spring.factories]", var14);
}
}
}
- 项目资源:
META-INF/spring.factories
- 系统资源:
META-INF/spring.factories
- 从这些资源中配置了所有的nextElement(自动配置),分装成properties
3.11. spring.factories
自动配置根源。
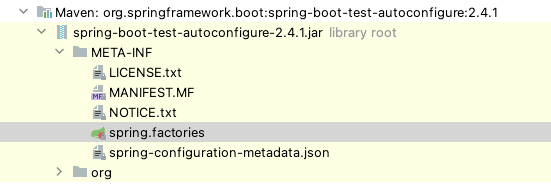
所以,自动配置真正实现是从classpath中搜寻所有的META-INF/spring.factories
配置文件 ,并将其中对应的 org.springframework.boot.autoconfigure
. 包下的配置项,通过反射实例化为对应标注了@Configuration
的JavaConfig形式的oC容器配置类 , 然后将这些都汇总成为一个实例并加载到IoC容器中。
4. 结论
- SpringBoot在启动的时候从类路径下的
META-INF/spring.factories
中获取EnableAutoConfiguration
指定的值 - 将这些值作为自动配置类导入容器 , 自动配置类就生效 , 帮我们进行自动配置工作;
- 以前我们需要自动配置的东西,现在springboot帮我们做了
- 整合JavaEE,整体解决方案和自动配置的东西都在
springboot-autoconfigure
的jar包中; - 它会把所有需要导入的组件,以类名的方式返回,这些组件就会被添加到容器中
- 它会给容器中导入非常多的自动配置类 (xxxAutoConfiguration), 就是给容器中导入这个场景需要的所有组件 , 并自动配置,@Configuration(javaConfig)
- 有了自动配置类 , 免去了我们手动编写配置注入功能组件等的工作
5. 回到主程序
SpringApplication.run主要分成两部分:
- 一是SpringApplication的实例化,
- 二是run方法的执行
5.1. SpringApplication
public SpringApplication(ResourceLoader resourceLoader, Class... primarySources) {
// ......
this.webApplicationType = WebApplicationType.deduceFromClasspath();
this.setInitializers(this.getSpringFactoriesInstances();
this.setListeners(this.getSpringFactoriesInstances(ApplicationListener.class));
this.mainApplicationClass = this.deduceMainApplicationClass();
}
这个类主要做了以下四件事情:
推断应用的类型是普通的项目还是Web项目
查找并加载所有可用初始化器 , 设置到initializers属性中
找出所有的应用程序监听器,设置到listeners属性中
推断并设置main方法的定义类,找到运行的主类
6. 自动配置原理
SpringBoot启动的时候加载主配置类,开启了自动配置功能 @EnableAutoConfiguration
@EnableAutoConfiguration 作用
- 利用EnableAutoConfigurationImportSelector给容器中导入一些组件
- 可以查看selectImports()方法的内容,他返回了一个autoConfigurationEnty,来自
this.getAutoConfigurationEntry(autoConfigurationMetadata,annotationMetadata);
这个方法我们继续来跟踪: 这个方法有一个值:
List<String> configurations = getCandidateConfigurations(annotationMetadata, attributes);
叫做获取候选的配置 ,我们点击继续跟踪SpringFactoriesLoader.loadFactoryNames()
- 扫描所有jar包类路径下
META-INF/spring.factories
- 把扫描到的这些文件的内容包装成properties对象
- 从properties中获取到EnableAutoConfiguration.class类(类名)对应的值,然后把他们添加在容器中
- 在类路径下,
META-INF/spring.factories
里面配置的所有EnableAutoConfiguration的值加入到容器中
每一个xxxAutoConfiguration类都是容器中的一个组件,都加入到容器中,用他们来做自动配置。
每一个自动配置类进行自动配置功能。
以HttpEncodingAutoConfiguration(Http编码自动配置)为例解释自动配置原理。
// 表示这是一个配置类,和以前编写的配置文件一样,也可以给容器中添加组件 @Configuration // 启动指定类的ConfigurationProperties功能 // 进入这个HttpProperties查看,将配置文件中对应的值和HttpProperties绑定起来 // 并把HttpProperties加入到ioc容器中 @EnableConfigurationProperties({HttpProperties.class}) // Spring底层@Conditional注解 // 根据不同的条件判断,如果满足指定的条件,整个配置类里面的配置就会生效; // 这里的意思就是判断当前应用是否是web应用,如果是,当前配置类生效 @ConditionalOnWebApplication( type = Type.SERVLET ) // 判断当前项目有没有这个类CharacterEncodingFilter;SpringMVC中进行乱码解决的过滤器; @ConditionalOnClass({CharacterEncodingFilter.class}) // 判断配置文件中是否存在某个配置:spring.http.encoding.enabled // 如果不存在,判断也是成立的 // 即使我们配置文件中不配置spring.http.encoding.enabled=true,也是默认生效的 @ConditionalOnProperty( prefix = "spring.http.encoding", value = {"enabled"}, matchIfMissing = true ) public class HttpEncodingAutoConfiguration { // 这里和SpringBoot的配置文件映射了 private final Encoding properties; // 只有一个有参构造器的情况下,参数的值就会从容器中拿 public HttpEncodingAutoConfiguration(HttpProperties properties) { this.properties = properties.getEncoding(); } // 给容器中添加一个组件,这个组件的某些值需要从properties中获取 @Bean // 判断容器没有这个组件 @ConditionalOnMissingBean public CharacterEncodingFilter characterEncodingFilter() { CharacterEncodingFilter filter = new OrderedCharacterEncodingFilter(); filter.setEncoding(this.properties.getCharset().name()); filter.setForceRequestEncoding(this.properties.shouldForce(org.springframework.boot.autoconfigure.http.HttpProperties.Encoding.Type.REQUEST)); filter.setForceResponseEncoding(this.properties.shouldForce(org.springframework.boot.autoconfigure.http.HttpProperties.Encoding.Type.RESPONSE)); return filter; } // ... }
总结:
根据当前不同的条件判断,决定这个配置类是否生效。
一但这个配置类生效;这个配置类就会给容器中添加各种组件。
这些组件的属性是从对应的properties类中获取的,这些类里面的每一个属性又是和配置文件绑定的。
所有在配置文件中能配置的属性都是在xxxxProperties类中封装的。
配置文件能配置什么就可以参照某个功能对应的这个属性类。
//从配置文件中获取指定的值和bean的属性进行绑定
@ConfigurationProperties(prefix = "spring.http")
public class HttpProperties {
// .....
}
SpringBoot启动会加载大量的自动配置类。
只需要看需要的功能有没有在SpringBoot默认写好的自动配置类当中。
我们再来看这个自动配置类中到底配置了哪些组件(只要我们要用的组件存在在其中,我们就不需要再手动配置了)。
给容器中自动配置类添加组件的时候,会从properties类中获取某些属性。我们只需要在配置文件中指定这些属性的值即可。
xxxxAutoConfigurartion:自动配置类。给容器中添加组件
xxxxProperties:封装配置文件中相关属性。
7. @Conditional
作用:必须是@Conditional指定的条件成立,才给容器中添加组件,配置配里面的所有内容才生效。
@Conditional扩展注解 | 作用(判断是否满足当前指定条件) |
---|---|
@ConditionalOnJava | 系统的java版本是否符合要求 |
@ConditionalOnJava | 容器中存在指定Bean ; |
@ConditionalOnMissingBean | 容器中不存在指定Bean ; |
@ConditionalOnExpression | 满足SpEL表达式指定 |
@ConditionalOnClass | 系统中有指定的类 |
@ConditionalOnMissingClass | 系统中没有指定的类 |
@ConditionalOnSingleCandidate | 容器中只有一个指定的Bean ,或者这个Bean是首选Bean |
@ConditionalOnProperty | 系统中指定的属性是否有指定的值 |
@ConditionalOnResource | 类路径下是否存在指定资源文件 |
@ConditionalOnWebApplication | 当前是web环境 |
@ConditionalOnNotWebApplication | 当前不是web环境 |
@ConditionalOnJndi | JNDI存在指定项 |
那么多的自动配置类,必须在一定的条件下才能生效;也就是说,我们加载了这么多的配置类,但不是所有的都生效了。
8. 查看自动配置类是否生效
可以在application.properties通过启用 debug=true
属性;
在控制台打印自动配置报告,这样我们就可以很方便的知道哪些自动配置类生效;
debug=true
Positive matches:(自动配置类启用的:正匹配)
Negative matches:(没有启动,没有匹配成功的自动配置类:负匹配)
Unconditional classes: (没有条件的类)